Integrating google maps in rails without gmap4rails gem
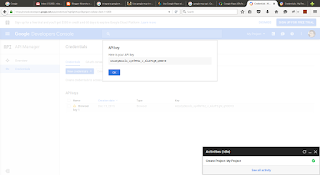
To Integrate google maps in rails application without gem 1.) Go to the google maps https://developers.google.com/maps/web/ 2.) Click Get a key 3.) Go to the page where you want to show the google maps and add this tag <script src="https://maps.googleapis.com/maps/api/js?v=3&key=' YOURAPIKEY '®ion=US" type="text/javascript"></script> 4.) Create a div for map <div id="map-container" style='width: 360px; height: 400px;'> <div id="map-canvas"></div> </div> 5.) Now what you need is latitude and longitude for the marker to show for that in rails you need to use a gem " Gecoder " ( https://github.com/alexreisner/geocoder ) 6.) In your Model here is School geocoded_by :address after_validation :geocode attr_accessor :address, :latitu...